This article discusses how you can leverage Create user (/rest/user) Rest API endpoint to create bulk users in Jira. This approach is targeted for all the Jira versions, where the /rest/api/2/user endpoint is supported.
Prior to the script execution, the CSV file with the user information or supported payload(emailAddress, displayName, name, password) is to be prepared.
Option 1: Using Bash command line
Prepare and create the script as below(by replacing Jira base URL, API username, password and path to CSV file):
|
Now, create the CSV file with user information:
# cat test.csv
test1,Test User 1,test1@sample.com,Passw0rd1
test2,Test User 2,test2@sample.com,Passw0rd2
test3,Test User 3,test3@sample.com,Passw0rd3
test4,Test User 4,test4@sample.com,Passw0rd4
test5,Test User 5,test5@sample.com,Passw0rd5
bulkuser.sh
) to create users in bulk.import
sys
import
csv
import
os
from optparse
import
OptionParser
import
requests
from requests.auth
import
HTTPBasicAuth
import
json
parser = OptionParser()
parser.add_option(
"--username"
, dest=
"username"
, help=
"API username"
)
parser.add_option(
"--password"
, dest=
"password"
, help=
"API password"
)
parser.add_option(
"--url"
, dest=
"weburl"
, help=
"Endpoint hostname"
)
parser.add_option(
"--input-file"
, dest=
"INPUT_FILE"
, help=
"CSV values of emailAddress name displayName"
)
parser.add_option(
"--csv-delimiter"
, dest=
"dlimit"
, help=
"CSV seperator used"
)
(options, args) = parser.parse_args()
if
not options.INPUT_FILE:
parser.error(
"INPUT_FILE must be specified"
)
if
not options.username or not options.weburl or not options.password or not options.dlimit :
parser.error(
"--username <username> --password <password> --url <endpoint uri> --input-file <input csv file with header emailAddress name displayName> --csv-delimiter <; or ,>"
)
url =
"https://"
+options.weburl+
"/rest/api/2/user"
auth = HTTPBasicAuth(options.username, options.password)
headers = {
"Accept"
:
"application/json"
,
"Content-Type"
:
"application/json"
}
with
open
(options.INPUT_FILE) as csvfile:
reader = csv.DictReader(csvfile, delimiter=options.dlimit)
for
row
in
reader:
emailAddress = row[
'emailAddress'
]
displayName = row[
'displayName'
]
name = row[
'name'
]
password = row[
'password'
]
payload = json.dumps( {
"emailAddress"
: emailAddress,
"displayName"
: displayName,
"name"
: name,
"password"
: password } )
response = requests.request(
"POST"
, url, data=payload, headers=headers, auth=auth)
print(json.dumps(json.loads(response.text), sort_keys=True, indent=4, separators=(
","
,
": "
)))
# cat bulkuser.py
name,displayName,emailAddress,password
test31,Test User 31,123@sample.com,Passw0rd1
test32,Test User 32,123@sample.com,Passw0rd2
test33,Test User 33,123@sample.com,Passw0rd3
test34,Test User 34,123@sample.com,Passw0rd4
test35,Test User 35,123@sample.com,Passw0rd5
python bulkuser.py --username admin --password admin --url jira.atlassian.com --input-file ./test.csv --csv-delimiter ,
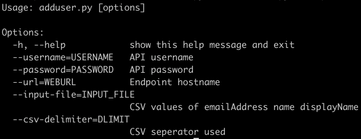
Ranjith Koolath
Online forums and learning are now in one easy-to-use experience.
By continuing, you accept the updated Community Terms of Use and acknowledge the Privacy Policy. Your public name, photo, and achievements may be publicly visible and available in search engines.
1 comment